2021-09-27 23:32:09 +08:00
[](http://commitizen.github.io/cz-cli/) 
2016-03-15 02:15:01 +08:00
2021-09-27 23:32:09 +08:00
# Awesome Cordova Plugins
2015-11-28 13:05:15 +08:00
2021-09-27 23:32:09 +08:00
Awesome Cordova Plugins is a curated set of wrappers for Cordova plugins that make adding any native functionality you need to your [Ionic ](https://ionicframework.com/ ) mobile app easy.
2016-02-19 01:54:23 +08:00
2021-09-27 23:32:09 +08:00
Awesome Cordova Plugins wraps plugin callbacks in a Promise or Observable, providing a common interface for all plugins and making it easy to use plugins with Angular change detection.
2017-03-22 01:55:10 +08:00
2021-09-27 23:30:46 +08:00
To learn more about the reasons why Ionic Native was renamed to Awesome Cordova Plugins, read the official [Ionic blog ](https://ionicframework.com/blog/a-new-chapter-for-ionic-native/ ) post by Max Lyncht.
2020-06-09 23:38:08 +08:00
2020-04-10 22:12:27 +08:00
## Capacitor Support
2022-12-31 16:04:59 +08:00
In addition to Cordova, Awesome Cordova Plugins also works with [Capacitor ](https://capacitorjs.com ), Ionic's official native runtime. Basic usage below. For complete details, [see the Capacitor documentation ](https://capacitorjs.com/docs/v2/cordova/using-cordova-plugins ).
2020-04-10 22:12:27 +08:00
2017-03-21 04:51:33 +08:00
## Installation
2021-09-27 23:32:09 +08:00
Run following command to install Awesome Cordova Plugins in your project.
2018-08-17 04:43:50 +08:00
2017-05-01 01:19:55 +08:00
```bash
2021-09-27 23:32:09 +08:00
npm install @awesome -cordova-plugins/core --save
2017-03-21 04:51:33 +08:00
```
2021-09-27 23:32:09 +08:00
You also need to install the Awesome Cordova Plugins package for each plugin you want to add. Please see the [Awesome Cordova Plugins documentation ](https://ionicframework.com/docs/native/ ) for complete instructions on how to add and use the plugins.
2017-03-21 04:51:33 +08:00
## Documentation
2016-05-10 00:01:51 +08:00
2021-09-27 23:32:09 +08:00
For the full Awesome Cordova Plugins documentation, please visit [https://ionicframework.com/docs/native/ ](https://ionicframework.com/docs/native/ ).
2016-05-10 00:01:51 +08:00
2017-03-22 01:55:10 +08:00
### Basic Usage
2016-02-19 01:54:23 +08:00
2017-12-29 23:47:34 +08:00
#### Ionic/Angular apps
2020-05-16 20:40:49 +08:00
To use a plugin, import and add the plugin provider to your `@NgModule` , and then inject it where you wish to use it.
2017-12-29 23:40:46 +08:00
Make sure to import the injectable class from the `/ngx` directory as shown in the following examples:
2016-02-19 01:54:23 +08:00
2017-03-21 04:46:29 +08:00
```typescript
2017-03-22 01:55:10 +08:00
// app.module.ts
2021-09-27 23:32:09 +08:00
import { Camera } from '@awesome-cordova-plugins/camera/ngx';
2017-03-22 01:55:10 +08:00
...
@NgModule ({
...
providers: [
...
Camera
...
]
...
})
export class AppModule { }
2017-03-22 04:50:08 +08:00
```
2017-03-22 01:55:10 +08:00
2017-03-22 04:50:08 +08:00
```typescript
2021-09-27 23:32:09 +08:00
import { Geolocation } from '@awesome-cordova-plugins/geolocation/ngx';
2017-03-21 04:46:08 +08:00
import { Platform } from 'ionic-angular';
@Component ({ ... })
export class MyComponent {
2017-09-11 21:56:07 +08:00
constructor(private geolocation: Geolocation, private platform: Platform) {
2017-03-22 01:55:10 +08:00
2018-07-02 16:59:40 +08:00
this.platform.ready().then(() => {
2017-03-22 01:55:10 +08:00
2017-03-21 04:46:08 +08:00
// get position
2018-07-02 16:59:40 +08:00
this.geolocation.getCurrentPosition().then(pos => {
2017-03-21 04:46:08 +08:00
console.log(`lat: ${pos.coords.latitude}, lon: ${pos.coords.longitude}`)
2016-08-02 03:25:55 +08:00
});
2017-03-22 01:55:10 +08:00
2017-03-21 04:46:08 +08:00
// watch position
const watch = geolocation.watchPosition().subscribe(pos => {
console.log(`lat: ${pos.coords.latitude}, lon: ${pos.coords.longitude}`)
});
2017-03-22 01:55:10 +08:00
2017-03-21 04:46:08 +08:00
// to stop watching
watch.unsubscribe();
2016-08-02 03:25:55 +08:00
});
2018-08-17 04:43:50 +08:00
2017-03-21 04:46:08 +08:00
}
2018-08-17 04:43:50 +08:00
2017-03-21 04:46:08 +08:00
}
2016-08-02 03:25:55 +08:00
```
2020-04-10 22:12:27 +08:00
#### Ionic/React apps
2021-09-27 23:32:09 +08:00
React apps must use Capacitor to build native mobile apps. However, Awesome Cordova Plugins (and therefore, Cordova plugins) can still be used.
2020-04-10 22:12:27 +08:00
```bash
# Install Core library (once per project)
2021-09-27 23:32:09 +08:00
npm install @awesome -cordova-plugins/core
2020-04-10 22:12:27 +08:00
2021-09-27 23:32:09 +08:00
# Install Awesome Cordova Plugins TypeScript wrapper
npm install @awesome -cordova-plugins/barcode-scanner
2020-04-10 22:12:27 +08:00
# Install Cordova plugin
npm install phonegap-plugin-barcodescanner
# Update native platform project(s) to include newly added plugin
ionic cap sync
```
2020-05-16 20:40:49 +08:00
Import the plugin object then use its static methods:
2020-04-10 22:12:27 +08:00
```typescript
2021-09-27 23:32:09 +08:00
import { BarcodeScanner } from '@awesome-cordova-plugins/barcode-scanner';
2020-04-10 22:12:27 +08:00
const Tab1: React.FC = () => {
const openScanner = async () => {
const data = await BarcodeScanner.scan();
console.log(`Barcode data: ${data.text}`);
};
return (
< IonPage >
< IonHeader >
< IonToolbar >
< IonTitle > Tab 1< / IonTitle >
< / IonToolbar >
< / IonHeader >
< IonContent >
< IonButton onClick = {openScanner} > Scan barcode< / IonButton >
< / IonContent >
< / IonPage >
);
};
```
2017-12-29 23:47:34 +08:00
#### ES2015+/TypeScript
2020-05-16 20:40:49 +08:00
2017-12-29 23:40:46 +08:00
These modules can work in any ES2015+/TypeScript app (including Angular/Ionic apps). To use any plugin, import the class from the appropriate package, and use it's static methods.
2020-05-16 20:40:49 +08:00
2017-12-29 23:40:46 +08:00
```js
2021-09-27 23:32:09 +08:00
import { Camera } from '@awesome-cordova-plugins/camera';
2017-12-29 23:40:46 +08:00
document.addEventListener('deviceready', () => {
Camera.getPicture()
2021-09-28 04:09:05 +08:00
.then((data) => console.log('Took a picture!', data))
.catch((e) => console.log('Error occurred while taking a picture', e));
2017-12-29 23:40:46 +08:00
});
```
2017-03-22 02:27:42 +08:00
2017-12-29 23:40:46 +08:00
#### AngularJS
2020-05-16 20:40:49 +08:00
2021-09-27 23:32:09 +08:00
Awesome Cordova Plugins generates an AngularJS module in runtime and prepares a service for each plugin. To use the plugins in your AngularJS app:
2020-05-16 20:40:49 +08:00
2021-09-27 23:32:09 +08:00
1. Download the latest bundle from the [Github releases ](https://github.com/danielsogl/awesome-cordova-plugins/releases ) page.
2017-12-29 23:40:46 +08:00
2. Include it in `index.html` before your app's code.
3. Inject `ionic.native` module in your app.
4. Inject any plugin you would like to use with a `$cordova` prefix.
```js
2020-05-16 20:40:49 +08:00
angular.module('myApp', ['ionic.native']).controller('MyPageController', function ($cordovaCamera) {
$cordovaCamera.getPicture().then(
function (data) {
console.log('Took a picture!', data);
},
function (err) {
console.log('Error occurred while taking a picture', err);
}
);
});
2017-12-29 23:40:46 +08:00
```
#### Vanilla JS
2020-05-16 20:40:49 +08:00
2021-09-27 23:32:09 +08:00
To use Awesome Cordova Plugins in any other setup:
2020-05-16 20:40:49 +08:00
2021-09-27 23:32:09 +08:00
1. Download the latest bundle from the [Github releases ](https://github.com/danielsogl/awesome-cordova-plugins/releases ) page.
2017-12-29 23:40:46 +08:00
2. Include it in `index.html` before your app's code.
3. Access any plugin using the global `IonicNative` variable.
```js
2020-05-16 20:40:49 +08:00
document.addEventListener('deviceready', function () {
IonicNative.Camera.getPicture().then(
function (data) {
console.log('Took a picture!', data);
},
function (err) {
console.log('Error occurred while taking a picture', err);
}
);
2017-12-29 23:40:46 +08:00
});
```
2017-12-29 23:47:34 +08:00
### Mocking and Browser Development (Ionic/Angular apps only)
2017-12-29 23:40:46 +08:00
2021-09-27 23:32:09 +08:00
Awesome Cordova Plugins makes it possible to mock plugins and develop nearly the entirety of your app in the browser or in `ionic serve` .
2017-03-22 02:27:42 +08:00
To do this, you need to provide a mock implementation of the plugins you wish to use. Here's an example of mocking the `Camera` plugin to return a stock image while in development:
First import the `Camera` class in your `src/app/app.module.ts` file:
2017-05-01 01:19:55 +08:00
```typescript
2021-09-27 23:32:09 +08:00
import { Camera } from '@awesome-cordova-plugins/camera/ngx';
2017-03-22 02:27:42 +08:00
```
Then create a new class that extends the `Camera` class with a mock implementation:
2017-05-01 01:19:55 +08:00
```typescript
2017-03-22 02:27:42 +08:00
class CameraMock extends Camera {
getPicture(options) {
return new Promise((resolve, reject) => {
2018-08-17 04:43:50 +08:00
resolve('BASE_64_ENCODED_DATA_GOES_HERE');
});
2017-03-22 02:27:42 +08:00
}
}
```
Finally, override the previous `Camera` class in your `providers` for this module:
2017-05-01 01:19:55 +08:00
```typescript
2018-08-17 04:43:50 +08:00
providers: [{ provide: Camera, useClass: CameraMock }];
2017-03-22 02:27:42 +08:00
```
Here's the full example:
2017-05-01 01:19:55 +08:00
```typescript
2018-07-02 16:59:40 +08:00
import { ErrorHandler, NgModule } from '@angular/core';
2017-05-01 01:19:55 +08:00
import { BrowserModule } from '@angular/platform-browser';
2017-03-22 02:27:42 +08:00
import { IonicApp, IonicModule, IonicErrorHandler } from 'ionic-angular';
import { MyApp } from './app.component';
import { HomePage } from '../pages/home/home';
2021-09-27 23:32:09 +08:00
import { Camera } from '@awesome-cordova-plugins/camera/ngx';
2017-03-22 02:27:42 +08:00
2018-07-02 16:59:40 +08:00
import { HomePage } from '../pages/home/home';
import { MyApp } from './app.component';
2017-03-22 02:27:42 +08:00
class CameraMock extends Camera {
getPicture(options) {
return new Promise((resolve, reject) => {
2018-07-02 16:59:40 +08:00
resolve('BASE_64_ENCODED_DATA_GOES_HERE');
});
2017-03-22 02:27:42 +08:00
}
}
@NgModule ({
2018-08-17 04:43:50 +08:00
declarations: [MyApp, HomePage],
imports: [BrowserModule, IonicModule.forRoot(MyApp)],
2017-03-22 02:27:42 +08:00
bootstrap: [IonicApp],
2018-08-17 04:43:50 +08:00
entryComponents: [MyApp, HomePage],
2017-03-22 02:27:42 +08:00
providers: [
2017-12-29 23:40:46 +08:00
{ provide: ErrorHandler, useClass: IonicErrorHandler },
2020-05-16 20:40:49 +08:00
{ provide: Camera, useClass: CameraMock },
],
2017-03-22 02:27:42 +08:00
})
export class AppModule {}
```
2016-02-19 01:54:23 +08:00
### Runtime Diagnostics
2021-09-27 23:32:09 +08:00
Spent way too long diagnosing an issue only to realize a plugin wasn't firing or installed? Awesome Cordova Plugins lets you know what the issue is and how you can resolve it.
2016-02-19 01:54:23 +08:00
2017-05-14 10:46:09 +08:00
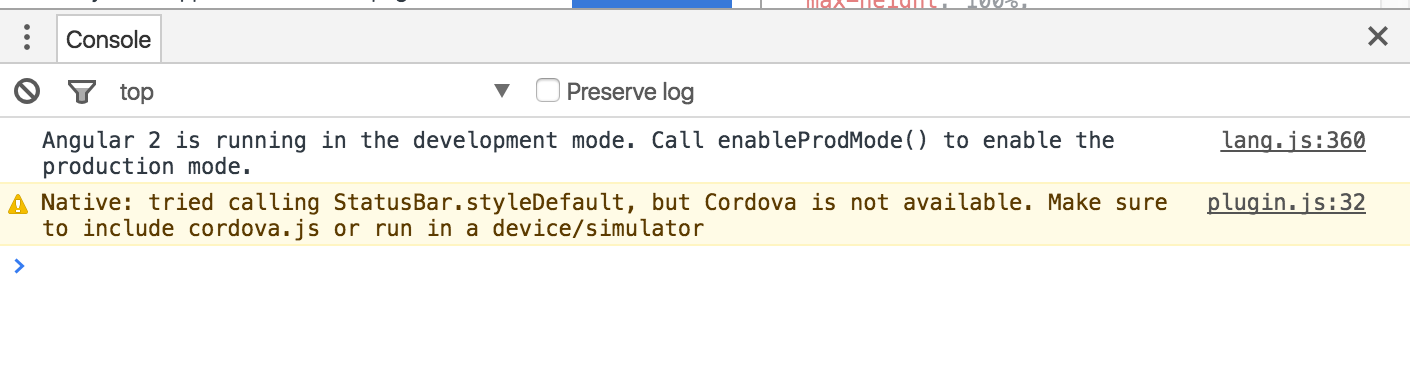
2016-07-27 02:34:53 +08:00
2016-02-27 01:31:21 +08:00
## Plugin Missing?
2018-08-17 04:43:50 +08:00
2021-09-27 23:32:09 +08:00
Let us know or submit a PR! Take a look at [the Developer Guide ](https://github.com/danielsogl/awesome-cordova-plugins/blob/master/DEVELOPER.md ) for more on how to contribute. :heart:
2016-02-27 01:31:21 +08:00
2015-11-29 08:36:38 +08:00
# Credits
2017-05-14 10:46:09 +08:00
Ibby Hadeed - [@ihadeed ](https://github.com/ihadeed )
2016-03-16 03:12:21 +08:00
2018-07-02 16:59:40 +08:00
Daniel Sogl - [@sogldaniel ](https://twitter.com/sogldaniel )
2017-05-14 10:46:09 +08:00
Tim Lancina - [@timlancina ](https://twitter.com/timlancina )
2016-02-11 00:07:19 +08:00
2017-03-22 01:55:10 +08:00
Mike Hartington - [@mhartington ](https://twitter.com/mhartington )
2017-05-14 10:46:09 +08:00
Max Lynch - [@maxlynch ](https://twitter.com/maxlynch )
2015-11-29 08:36:38 +08:00
Rob Wormald - [@robwormald ](https://twitter.com/robwormald )