mirror of
https://github.com/apache/cordova-android.git
synced 2025-01-19 07:02:51 +08:00
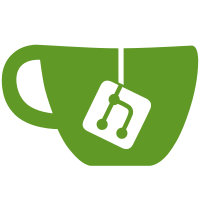
* feat!: Upgrade to Gradle and AGP 8 * java 17 * feat!: API 34 Support API 34: Upgrade AGP from 8.2.0-rc01 to 8.2.0-rc02 API 34: Upgrade AGP from 8.2.0-rc02 to 8.2.0-rc03 API 34: Upgrade AGP from 8.2.0-rc03 to 8.2.0 feat: add AndroidKotlinJVMTarget preference to set the kotlin JVM target This is in addition to the java source / target compatibility preferences. AndroidKotlinJVMTarget is only affective if Kotlin is enabled. chore: Upgrade Gradle from 8.4 to 8.5 AGP 8.2.0 -> 8.2.1 Gradle 8.5 -> 8.7 fix: Add --validate-url to gradle wrapper commands AGP 8.4.0 * fix(test): ProjectBuilder using Gradle 8.3, no longer supported version * API 34: Change Kotlin JVM Target default. The new default value is null. When null, it will by default to the Java Target compatibility. Updating AndroidJavaTargetCompatibility will also influence the Kotlin JVM target, unless if AndroidKotlinJVMTarget is also explicitly defined. * removed leftover debug prints * API 34: Gradle Wrapper * API 34: ratignore generated gradle wrapper files * fix gradle wrapper jar via git attributes * fix(test): normalise gradle paths * fix(windows): Gradle paths * fix(windows): Keep CRLF endings for bat files * chore: Updated license for Gradle Wrapper 8.7 pointer * API 34 Support Gradle Tools project * API 34: omit --validate-url on installing the wrapper * revert: LICENSE notice on bundling the gradle wrapper jar * Revert: AGP 8.4 -> 8.3 * test(ci): Added NodeJS 22 to the test matrix --------- Co-authored-by: jcesarmobile <jcesarmobile@gmail.com>
375 lines
14 KiB
JavaScript
375 lines
14 KiB
JavaScript
/*
|
|
Licensed to the Apache Software Foundation (ASF) under one
|
|
or more contributor license agreements. See the NOTICE file
|
|
distributed with this work for additional information
|
|
regarding copyright ownership. The ASF licenses this file
|
|
to you under the Apache License, Version 2.0 (the
|
|
"License"); you may not use this file except in compliance
|
|
with the License. You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing,
|
|
software distributed under the License is distributed on an
|
|
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
|
|
KIND, either express or implied. See the License for the
|
|
specific language governing permissions and limitations
|
|
under the License.
|
|
*/
|
|
|
|
const fs = require('fs-extra');
|
|
const path = require('path');
|
|
const execa = require('execa');
|
|
const glob = require('fast-glob');
|
|
const events = require('cordova-common').events;
|
|
const CordovaError = require('cordova-common').CordovaError;
|
|
const check_reqs = require('../check_reqs');
|
|
const PackageType = require('../PackageType');
|
|
const { compareByAll, isWindows } = require('../utils');
|
|
const { createEditor } = require('properties-parser');
|
|
const CordovaGradleConfigParserFactory = require('../config/CordovaGradleConfigParserFactory');
|
|
|
|
const MARKER = 'YOUR CHANGES WILL BE ERASED!';
|
|
const SIGNING_PROPERTIES = '-signing.properties';
|
|
const TEMPLATE =
|
|
'# This file is automatically generated.\n' +
|
|
'# Do not modify this file -- ' + MARKER + '\n';
|
|
|
|
const isPathArchSpecific = p => /-x86|-arm/.test(path.basename(p));
|
|
|
|
const outputFileComparator = compareByAll([
|
|
// Sort arch specific builds after generic ones
|
|
isPathArchSpecific,
|
|
|
|
// Sort unsigned builds after signed ones
|
|
filePath => /-unsigned/.test(path.basename(filePath)),
|
|
|
|
// Sort by file modification time, latest first
|
|
filePath => -fs.statSync(filePath).mtime.getTime(),
|
|
|
|
// Sort by file name length, ascending
|
|
filePath => filePath.length
|
|
]);
|
|
|
|
/**
|
|
* @param {'apk' | 'aab'} bundleType
|
|
* @param {'debug' | 'release'} buildType
|
|
* @param {{arch?: string}} options
|
|
*/
|
|
function findOutputFiles (bundleType, buildType, { arch } = {}) {
|
|
let files = glob.sync(`**/*.${bundleType}`, {
|
|
absolute: true,
|
|
cwd: path.resolve(this[`${bundleType}Dir`], buildType)
|
|
}).map(path.normalize);
|
|
|
|
if (files.length === 0) return files;
|
|
|
|
// Assume arch-specific build if newest apk has -x86 or -arm.
|
|
const archSpecific = isPathArchSpecific(files[0]);
|
|
|
|
// And show only arch-specific ones (or non-arch-specific)
|
|
files = files.filter(p => isPathArchSpecific(p) === archSpecific);
|
|
|
|
if (archSpecific && files.length > 1 && arch) {
|
|
files = files.filter(p => path.basename(p).includes('-' + arch));
|
|
}
|
|
|
|
return files.sort(outputFileComparator);
|
|
}
|
|
|
|
class ProjectBuilder {
|
|
constructor (rootDirectory) {
|
|
this.root = rootDirectory;
|
|
this.apkDir = path.join(this.root, 'app', 'build', 'outputs', 'apk');
|
|
this.aabDir = path.join(this.root, 'app', 'build', 'outputs', 'bundle');
|
|
}
|
|
|
|
getArgs (cmd, opts) {
|
|
let args = [];
|
|
if (opts.extraArgs) {
|
|
args = args.concat(opts.extraArgs);
|
|
}
|
|
|
|
let buildCmd = cmd;
|
|
if (opts.packageType === PackageType.BUNDLE) {
|
|
if (cmd === 'release') {
|
|
buildCmd = ':app:bundleRelease';
|
|
} else if (cmd === 'debug') {
|
|
buildCmd = ':app:bundleDebug';
|
|
}
|
|
} else {
|
|
if (cmd === 'release') {
|
|
buildCmd = 'cdvBuildRelease';
|
|
} else if (cmd === 'debug') {
|
|
buildCmd = 'cdvBuildDebug';
|
|
}
|
|
|
|
if (opts.arch) {
|
|
args.push('-PcdvBuildArch=' + opts.arch);
|
|
}
|
|
}
|
|
|
|
args.push(buildCmd);
|
|
|
|
return args;
|
|
}
|
|
|
|
getGradleWrapperPath () {
|
|
let wrapper = path.join(this.root, 'tools', 'gradlew');
|
|
|
|
if (isWindows()) {
|
|
wrapper += '.bat';
|
|
}
|
|
|
|
return wrapper;
|
|
}
|
|
|
|
/**
|
|
* Installs/updates the gradle wrapper
|
|
* @param {string} gradleVersion The gradle version to install. Ignored if CORDOVA_ANDROID_GRADLE_DISTRIBUTION_URL environment variable is defined
|
|
* @returns {Promise<void>}
|
|
*/
|
|
async installGradleWrapper (gradleVersion) {
|
|
if (process.env.CORDOVA_ANDROID_GRADLE_DISTRIBUTION_URL) {
|
|
events.emit('verbose', `Overriding Gradle Version via CORDOVA_ANDROID_GRADLE_DISTRIBUTION_URL (${process.env.CORDOVA_ANDROID_GRADLE_DISTRIBUTION_URL})`);
|
|
await execa('gradle', ['-p', path.join(this.root, 'tools'), 'wrapper', '--gradle-distribution-url', process.env.CORDOVA_ANDROID_GRADLE_DISTRIBUTION_URL], { stdio: 'inherit' });
|
|
} else {
|
|
await execa('gradle', ['-p', path.join(this.root, 'tools'), 'wrapper', '--gradle-version', gradleVersion], { stdio: 'inherit' });
|
|
}
|
|
}
|
|
|
|
readProjectProperties () {
|
|
function findAllUniq (data, r) {
|
|
const s = {};
|
|
let m;
|
|
while ((m = r.exec(data))) {
|
|
s[m[1]] = 1;
|
|
}
|
|
return Object.keys(s);
|
|
}
|
|
|
|
const data = fs.readFileSync(path.join(this.root, 'project.properties'), 'utf8');
|
|
return {
|
|
libs: findAllUniq(data, /^\s*android\.library\.reference\.\d+=(.*)(?:\s|$)/mg),
|
|
gradleIncludes: findAllUniq(data, /^\s*cordova\.gradle\.include\.\d+=(.*)(?:\s|$)/mg),
|
|
systemLibs: findAllUniq(data, /^\s*cordova\.system\.library\.\d+=((?!.*\().*)(?:\s|$)/mg),
|
|
bomPlatforms: findAllUniq(data, /^\s*cordova\.system\.library\.\d+=platform\((?:'|")(.*)(?:'|")\)/mg)
|
|
};
|
|
}
|
|
|
|
// Makes the project buildable, minus the gradle wrapper.
|
|
prepBuildFiles () {
|
|
// Update the version of build.gradle in each dependent library.
|
|
const pluginBuildGradle = path.join(__dirname, 'plugin-build.gradle');
|
|
const propertiesObj = this.readProjectProperties();
|
|
const subProjects = propertiesObj.libs;
|
|
|
|
// Check and copy the gradle file into the subproject
|
|
// Called by the loop before this function def
|
|
|
|
const checkAndCopy = function (subProject, root) {
|
|
const subProjectGradle = path.join(root, subProject, 'build.gradle');
|
|
// This is the future-proof way of checking if a file exists
|
|
// This must be synchronous to satisfy a Travis test
|
|
try {
|
|
fs.accessSync(subProjectGradle, fs.F_OK);
|
|
} catch (e) {
|
|
fs.copySync(pluginBuildGradle, subProjectGradle);
|
|
}
|
|
};
|
|
|
|
for (let i = 0; i < subProjects.length; ++i) {
|
|
if (subProjects[i] !== 'CordovaLib') {
|
|
checkAndCopy(subProjects[i], this.root);
|
|
}
|
|
}
|
|
|
|
// get project name cdv-gradle-config.
|
|
const cdvGradleConfig = CordovaGradleConfigParserFactory.create(this.root);
|
|
const projectName = cdvGradleConfig.getProjectNameFromPackageName();
|
|
|
|
// Remove the proj.id/name- prefix from projects: https://issues.apache.org/jira/browse/CB-9149
|
|
const settingsGradlePaths = subProjects.map(function (p) {
|
|
const realDir = p.replace(/[/\\]/g, ':');
|
|
const libName = realDir.replace(projectName + '-', '');
|
|
let str = 'include ":' + libName + '"\n';
|
|
if (realDir.indexOf(projectName + '-') !== -1) {
|
|
str += 'project(":' + libName + '").projectDir = new File("' + p + '")\n';
|
|
}
|
|
return str;
|
|
});
|
|
|
|
// Update subprojects within settings.gradle.
|
|
fs.writeFileSync(path.join(this.root, 'settings.gradle'),
|
|
'// GENERATED FILE - DO NOT EDIT\n' +
|
|
'apply from: "cdv-gradle-name.gradle"\n' +
|
|
'include ":"\n' +
|
|
settingsGradlePaths.join(''));
|
|
|
|
// Touch empty cdv-gradle-name.gradle file if missing.
|
|
if (!fs.pathExistsSync(path.join(this.root, 'cdv-gradle-name.gradle'))) {
|
|
fs.writeFileSync(path.join(this.root, 'cdv-gradle-name.gradle'), '');
|
|
}
|
|
|
|
// Update dependencies within build.gradle.
|
|
let buildGradle = fs.readFileSync(path.join(this.root, 'app', 'build.gradle'), 'utf8');
|
|
let depsList = '';
|
|
const root = this.root;
|
|
const insertExclude = function (p) {
|
|
const gradlePath = path.join(root, p, 'build.gradle');
|
|
const projectGradleFile = fs.readFileSync(gradlePath, 'utf-8');
|
|
if (projectGradleFile.indexOf('CordovaLib') !== -1) {
|
|
depsList += '{\n exclude module:("CordovaLib")\n }\n';
|
|
} else {
|
|
depsList += '\n';
|
|
}
|
|
};
|
|
subProjects.forEach(function (p) {
|
|
events.emit('log', 'Subproject Path: ' + p);
|
|
const libName = p.replace(/[/\\]/g, ':').replace(projectName + '-', '');
|
|
if (libName !== 'app') {
|
|
depsList += ' implementation(project(path: ":' + libName + '"))';
|
|
insertExclude(p);
|
|
}
|
|
});
|
|
// For why we do this mapping: https://issues.apache.org/jira/browse/CB-8390
|
|
const SYSTEM_LIBRARY_MAPPINGS = [
|
|
[/^\/?extras\/android\/support\/(.*)$/, 'com.android.support:support-$1:+'],
|
|
[/^\/?google\/google_play_services\/libproject\/google-play-services_lib\/?$/, 'com.google.android.gms:play-services:+']
|
|
];
|
|
|
|
propertiesObj.bomPlatforms.forEach(function (p) {
|
|
if (!/:.*:/.exec(p)) {
|
|
throw new CordovaError('Malformed BoM platform: ' + p);
|
|
}
|
|
|
|
// Add bom platform
|
|
depsList += ' implementation platform("' + p + '")\n';
|
|
});
|
|
|
|
propertiesObj.systemLibs.forEach(function (p) {
|
|
let mavenRef;
|
|
// It's already in gradle form if it has two ':'s
|
|
if (/:.*:/.exec(p)) {
|
|
mavenRef = p;
|
|
} else if (/:.*/.exec(p)) {
|
|
// Support BoM imports
|
|
mavenRef = p;
|
|
events.emit('warn', 'Library expects a BoM package: ' + p);
|
|
} else {
|
|
for (let i = 0; i < SYSTEM_LIBRARY_MAPPINGS.length; ++i) {
|
|
const pair = SYSTEM_LIBRARY_MAPPINGS[i];
|
|
if (pair[0].exec(p)) {
|
|
mavenRef = p.replace(pair[0], pair[1]);
|
|
break;
|
|
}
|
|
}
|
|
if (!mavenRef) {
|
|
throw new CordovaError('Unsupported system library (does not work with gradle): ' + p);
|
|
}
|
|
}
|
|
depsList += ' implementation "' + mavenRef + '"\n';
|
|
});
|
|
|
|
buildGradle = buildGradle.replace(/(SUB-PROJECT DEPENDENCIES START)[\s\S]*(\/\/ SUB-PROJECT DEPENDENCIES END)/, '$1\n' + depsList + ' $2');
|
|
let includeList = '';
|
|
|
|
propertiesObj.gradleIncludes.forEach(function (includePath) {
|
|
includeList += 'apply from: "../' + includePath + '"\n';
|
|
});
|
|
buildGradle = buildGradle.replace(/(PLUGIN GRADLE EXTENSIONS START)[\s\S]*(\/\/ PLUGIN GRADLE EXTENSIONS END)/, '$1\n' + includeList + '$2');
|
|
// This needs to be stored in the app gradle, not the root grade
|
|
fs.writeFileSync(path.join(this.root, 'app', 'build.gradle'), buildGradle);
|
|
}
|
|
|
|
prepEnv (opts) {
|
|
const self = this;
|
|
const config = this._getCordovaConfig();
|
|
return check_reqs.check_gradle()
|
|
.then(function () {
|
|
events.emit('verbose', `Using Gradle: ${config.GRADLE_VERSION}`);
|
|
return self.installGradleWrapper(config.GRADLE_VERSION);
|
|
}).then(function () {
|
|
return self.prepBuildFiles();
|
|
}).then(() => {
|
|
const signingPropertiesPath = path.join(self.root, `${opts.buildType}${SIGNING_PROPERTIES}`);
|
|
|
|
if (fs.existsSync(signingPropertiesPath)) fs.removeSync(signingPropertiesPath);
|
|
if (opts.packageInfo) {
|
|
fs.ensureFileSync(signingPropertiesPath);
|
|
const signingProperties = createEditor(signingPropertiesPath);
|
|
signingProperties.addHeadComment(TEMPLATE);
|
|
opts.packageInfo.appendToProperties(signingProperties);
|
|
}
|
|
});
|
|
}
|
|
|
|
/**
|
|
* @private
|
|
* @returns The user defined configs
|
|
*/
|
|
_getCordovaConfig () {
|
|
return fs.readJSONSync(path.join(this.root, 'cdv-gradle-config.json'));
|
|
}
|
|
|
|
/*
|
|
* Builds the project with gradle.
|
|
* Returns a promise.
|
|
*/
|
|
async build (opts) {
|
|
const wrapper = this.getGradleWrapperPath();
|
|
const args = this.getArgs(opts.buildType === 'debug' ? 'debug' : 'release', opts);
|
|
|
|
events.emit('verbose', `Running Gradle Build: ${wrapper} ${args.join(' ')}`);
|
|
|
|
try {
|
|
return await execa(wrapper, args, { stdio: 'inherit', cwd: path.resolve(this.root) });
|
|
} catch (error) {
|
|
if (error.toString().includes('failed to find target with hash string')) {
|
|
// Add hint from check_android_target to error message
|
|
try {
|
|
await check_reqs.check_android_target(this.root);
|
|
} catch (checkAndroidTargetError) {
|
|
error.message += '\n' + checkAndroidTargetError.message;
|
|
}
|
|
}
|
|
throw error;
|
|
}
|
|
}
|
|
|
|
clean (opts) {
|
|
const wrapper = this.getGradleWrapperPath();
|
|
const args = this.getArgs('clean', opts);
|
|
return execa(wrapper, args, { stdio: 'inherit', cwd: path.resolve(this.root) })
|
|
.then(() => {
|
|
fs.removeSync(path.join(this.root, 'out'));
|
|
|
|
['debug', 'release'].map(config => path.join(this.root, `${config}${SIGNING_PROPERTIES}`))
|
|
.forEach(file => {
|
|
const hasFile = fs.existsSync(file);
|
|
const hasMarker = hasFile && fs.readFileSync(file, 'utf8')
|
|
.includes(MARKER);
|
|
|
|
if (hasFile && hasMarker) fs.removeSync(file);
|
|
});
|
|
});
|
|
}
|
|
|
|
findOutputApks (build_type, arch) {
|
|
return findOutputFiles.call(this, 'apk', build_type, { arch });
|
|
}
|
|
|
|
findOutputBundles (build_type) {
|
|
return findOutputFiles.call(this, 'aab', build_type);
|
|
}
|
|
|
|
fetchBuildResults (build_type, arch) {
|
|
return {
|
|
apkPaths: this.findOutputApks(build_type, arch),
|
|
buildType: build_type
|
|
};
|
|
}
|
|
}
|
|
|
|
module.exports = ProjectBuilder;
|