mirror of
https://github.com/apache/cordova-android.git
synced 2025-01-31 09:02:50 +08:00
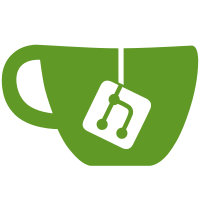
* fix: Gradle Args parsing * refactor: Applied ARGVParser.parseArgsStringToArgv -> parseArgsStringToArgv suggestion * test: Added deeper testing for gradle argument parsing
117 lines
3.7 KiB
JavaScript
117 lines
3.7 KiB
JavaScript
/*
|
|
Licensed to the Apache Software Foundation (ASF) under one
|
|
or more contributor license agreements. See the NOTICE file
|
|
distributed with this work for additional information
|
|
regarding copyright ownership. The ASF licenses this file
|
|
to you under the Apache License, Version 2.0 (the
|
|
"License"); you may not use this file except in compliance
|
|
with the License. You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing,
|
|
software distributed under the License is distributed on an
|
|
"AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
|
|
KIND, either express or implied. See the License for the
|
|
specific language governing permissions and limitations
|
|
under the License.
|
|
*/
|
|
|
|
const rewire = require('rewire');
|
|
const builders = require('../../lib/builders/builders');
|
|
|
|
describe('build', () => {
|
|
let build;
|
|
const builder = builders.getBuilder('FakeRootPath');
|
|
|
|
beforeEach(() => {
|
|
build = rewire('../../lib/build');
|
|
build.__set__({
|
|
events: jasmine.createSpyObj('eventsSpy', ['emit'])
|
|
});
|
|
|
|
// run needs `this` to behave like an Api instance
|
|
build.run = build.run.bind({
|
|
_builder: builder
|
|
});
|
|
|
|
spyOn(builder, 'build').and.returnValue(Promise.resolve({
|
|
paths: ['fake.apk'],
|
|
buildtype: 'debug'
|
|
}));
|
|
});
|
|
|
|
describe('argument parsing', () => {
|
|
let prepEnvSpy;
|
|
|
|
beforeEach(() => {
|
|
prepEnvSpy = spyOn(builder, 'prepEnv').and.returnValue(Promise.resolve());
|
|
});
|
|
|
|
describe('gradleArg', () => {
|
|
const baseOptions = {
|
|
packageType: 'apk',
|
|
arch: undefined,
|
|
prepEnv: undefined,
|
|
buildType: 'debug'
|
|
};
|
|
|
|
it('can parse single gradle argument', async () => {
|
|
await build.run({
|
|
argv: [
|
|
'node',
|
|
'--gradleArg=--stacktrace'
|
|
]
|
|
});
|
|
|
|
expect(prepEnvSpy).toHaveBeenCalledWith({
|
|
...baseOptions,
|
|
extraArgs: ['--stacktrace']
|
|
});
|
|
});
|
|
|
|
it('can parse multiple gradle arguments', async () => {
|
|
await build.run({
|
|
argv: [
|
|
'node',
|
|
'--gradleArg=--stacktrace --info'
|
|
]
|
|
});
|
|
|
|
expect(prepEnvSpy).toHaveBeenCalledWith({
|
|
...baseOptions,
|
|
extraArgs: ['--stacktrace', '--info']
|
|
});
|
|
});
|
|
|
|
it('can parse multiple gradle arguments with strings', async () => {
|
|
await build.run({
|
|
argv: [
|
|
'node',
|
|
'--gradleArg=--testArg="hello world"'
|
|
]
|
|
});
|
|
|
|
expect(prepEnvSpy).toHaveBeenCalledWith({
|
|
...baseOptions,
|
|
extraArgs: ['--testArg="hello world"']
|
|
});
|
|
});
|
|
|
|
it('gradle args will split when necessary', async () => {
|
|
await build.run({
|
|
argv: [
|
|
'node',
|
|
'--gradleArg=--warning-mode all'
|
|
]
|
|
});
|
|
|
|
expect(prepEnvSpy).toHaveBeenCalledWith({
|
|
...baseOptions,
|
|
extraArgs: ['--warning-mode', 'all']
|
|
});
|
|
});
|
|
});
|
|
});
|
|
});
|