mirror of
https://github.com/kubernetes/sample-controller.git
synced 2025-02-01 01:12:52 +08:00
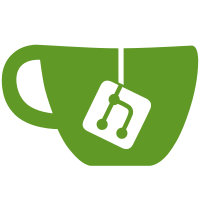
Automatic merge from submit-queue (batch tested with PRs 59965, 59115, 63076, 63059). If you want to cherry-pick this change to another branch, please follow the instructions <a href="https://github.com/kubernetes/community/blob/master/contributors/devel/cherry-picks.md">here</a>. Upgrade dep json-iterator/go to fix base64 decode bug **What this PR does / why we need it**: upgrade dep `json-iterator/go` to fix base64 decode bug #62742 **Which issue(s) this PR fixes** *(optional, in `fixes #<issue number>(, fixes #<issue_number>, ...)` format, will close the issue(s) when PR gets merged)*: Fixes #62742 **Special notes for your reviewer**: Just upgrade `json-iterator/go` to latest which includes base64 decode fix https://github.com/json-iterator/go/pull/266 No other code changes **Release note**: ```release-note None ``` Kubernetes-commit: 3dbcd1ddcee786f443f89a82514bbd9c6ad06c99
43 lines
956 B
Go
43 lines
956 B
Go
package jsoniter
|
|
|
|
import (
|
|
"io"
|
|
)
|
|
|
|
// IteratorPool a thread safe pool of iterators with same configuration
|
|
type IteratorPool interface {
|
|
BorrowIterator(data []byte) *Iterator
|
|
ReturnIterator(iter *Iterator)
|
|
}
|
|
|
|
// StreamPool a thread safe pool of streams with same configuration
|
|
type StreamPool interface {
|
|
BorrowStream(writer io.Writer) *Stream
|
|
ReturnStream(stream *Stream)
|
|
}
|
|
|
|
func (cfg *frozenConfig) BorrowStream(writer io.Writer) *Stream {
|
|
stream := cfg.streamPool.Get().(*Stream)
|
|
stream.Reset(writer)
|
|
return stream
|
|
}
|
|
|
|
func (cfg *frozenConfig) ReturnStream(stream *Stream) {
|
|
stream.out = nil
|
|
stream.Error = nil
|
|
stream.Attachment = nil
|
|
cfg.streamPool.Put(stream)
|
|
}
|
|
|
|
func (cfg *frozenConfig) BorrowIterator(data []byte) *Iterator {
|
|
iter := cfg.iteratorPool.Get().(*Iterator)
|
|
iter.ResetBytes(data)
|
|
return iter
|
|
}
|
|
|
|
func (cfg *frozenConfig) ReturnIterator(iter *Iterator) {
|
|
iter.Error = nil
|
|
iter.Attachment = nil
|
|
cfg.iteratorPool.Put(iter)
|
|
}
|